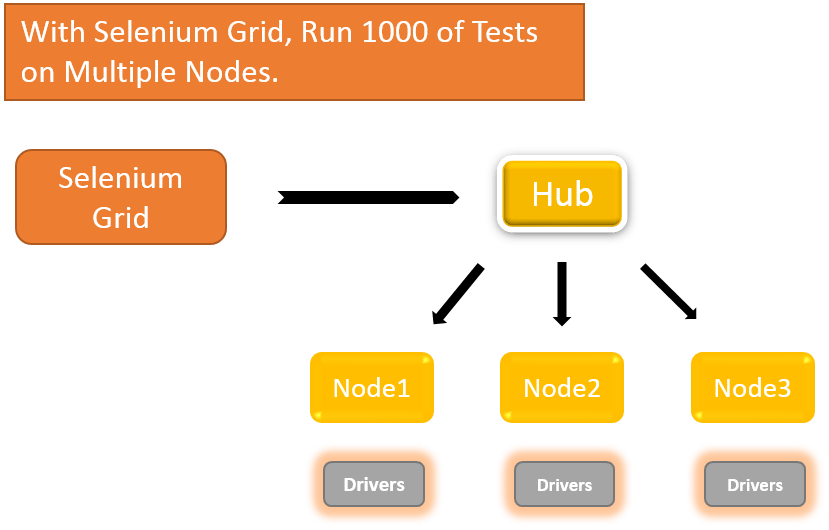
Selenium Grid has a Hub and a Node. Each hub may have many nodes.Selenium Grid helped me a lot in Compatibility check (Multiple Browsers & Multiple Platforms).This is grid helps us to execute multiple instances of WebDriver in parallel which uses the same code base (code which is present in only one system), hence the code need NOT be present on the other system they execute. The selenium-server-standalone includes Hub, WebDriver, and Selenium RC to execute the scripts in grid.
Hub – The hub can also be understood as a server which acts as the central point where the tests would be triggered. A Selenium Grid has only one Hub and it is launched on a single machine once.
How to start Hub : java -jar selenium-server-standalone-2.53.0.jar -role hub
Node – Nodes are the Selenium instances that are attached to the Hub which execute the tests. There can be one or more nodes in a grid which can be of any OS and can contain any of the Selenium supported browsers.
How to Start Node : java -jar selenium-server-standalone-2.53.0.jar -role node -hub http://192.168.xxxx:4444/grid/register -port 5556
There are few steps to be followed to use Selenium Grid
Step 1 :
Open command prompt and navigate to folder where the server is located. Run the server by using below command.
java -jar selenium-server-standalone-2.53.0.jar -role hub
The hub will use the port 4444 by default. This port can be changed by passing the different port number in command prompt provided the port is open and has not been assigned a task.
Now open browser and type : http://localhost:4444/grid/console.You will see Grid console.
Step 2 :
Go to the other machine where you intend to setup Nodes. Open the command prompt and run the below line by navigating to the dir where the standalone jar is present.
java -jar selenium-server-standalone-2.53.0.jar -role node -hub http://192.168.xxxx:4444/grid/register -port 5556
Step 3 : Now go to other node which has IE browser and IE driver, Open command prompt and type the below code.
C:\>java -Dwebdriver.ie.driver=D:\IEDriverServer.exe -jar D:\JAR\selenium-server-standalone-2.53.0.jar -role webdriver -hub http://192.169.xxxx:4444/grid/register -browser browserName=ie,platform=WINDOWS -port 5557
Step 4 : Now go to other node which has chromedriver and chrome browser.Type the below code.
C:\>java -Dwebdriver.chrome.driver=D:\chromedriver.exe -jar D:\JAR\selenium-server-standalone-2.53.0.jar -role webdriver -hub http://192.168.xxxx:4444/grid/register -browser browserName=chrome,platform=WINDOWS -port 5558
Now its time to write the code to execute your testcases in various nodes.Here i am using TestNG framework.Just create a class and paste the following code.
package seleniumGrid;
import java.net.MalformedURLException;
import java.net.URL;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.By;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.remote.DesiredCapabilities;
import org.openqa.selenium.remote.RemoteWebDriver;
import org.testng.annotations.AfterTest;
import org.testng.annotations.BeforeTest;
import org.testng.annotations.Parameters;
import org.testng.annotations.Test;
public class TestGridClass {
public WebDriver driver;
public String URL, Node;
protected ThreadLocal
@Parameters("browser")
@BeforeTest
public void launchbrowser(String browser) throws MalformedURLException {
String URL = "http://www.calculator.net";
if (browser.equalsIgnoreCase("firefox")) {
System.out.println(" Executing on FireFox");
String Node = "http://192.168.1.3:5556/wd/hub";
DesiredCapabilities cap = DesiredCapabilities.firefox();
cap.setBrowserName("firefox");
driver = new RemoteWebDriver(new URL(Node), cap);
// Puts an Implicit wait, Will wait for 10 seconds before throwing
// exception
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
// Launch website
driver.navigate().to(URL);
driver.manage().window().maximize();
}
else if (browser.equalsIgnoreCase("ie")) {
System.out.println(" Executing on IE");
DesiredCapabilities cap = DesiredCapabilities.chrome();
cap.setBrowserName("ie");
String Node = "http://192.168.1.3:5557/wd/hub";
driver = new RemoteWebDriver(new URL(Node), cap);
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
// Launch website
driver.navigate().to(URL);
driver.manage().window().maximize();
}
else if (browser.equalsIgnoreCase("chrome")) {
System.out.println(" Executing on CHROME");
DesiredCapabilities cap = DesiredCapabilities.chrome();
cap.setBrowserName("chrome");
String Node = "http://192.168.1.3:5558/wd/hub";
driver = new RemoteWebDriver(new URL(Node), cap);
driver.manage().timeouts().implicitlyWait(10, TimeUnit.SECONDS);
// Launch website
driver.navigate().to(URL);
driver.manage().window().maximize();
}
else {
throw new IllegalArgumentException("The Browser Type is Undefined");
}
}
@Test
public void calculatepercent() {
// Click on Math Calculators
driver.findElement(By.xpath("//a[contains(text(),'Math')]")).click();
// Click on Percent Calculators
driver.findElement(
By.xpath("//a[contains(text(),'Percentage Calculator')]"))
.click();
// Enter value 17 in the first number of the percent Calculator
driver.findElement(By.id("cpar1")).sendKeys("17");
// Enter value 35 in the second number of the percent Calculator
driver.findElement(By.id("cpar2")).sendKeys("35");
// Click Calculate Button
driver.findElement(
By.xpath("(//input[contains(@value,'Calculate')])[1]")).click();
// Get the Result Text based on its xpath
String result = driver.findElement(
By.xpath(".//*[@id='content']/p[2]/font/b")).getText();
// Print a Log In message to the screen
System.out.println(" The Result is " + result);
if (result.equals("5.95")) {
System.out.println(" The Result is Pass");
} else {
System.out.println(" The Result is Fail");
}
}
@AfterTest
public void closeBrowser() {
// driver.quit();
}
}
Now create a xml file and paste the following code
http://testng.org/testng-1.0.dtd
If you want to use 3 Firefox, then you should start the node using the <maxInstances> setting
java -jar selenium-server-standalone-2.53.0.jar -role webdriver -hub http://localhost:4444/grid/register -port 5556 -browser browserName=firefox,maxInstances=3
Conclusion :
- Selenium Grid is used to run multiple tests simultaneously in different browsers and platforms.
- To run test scripts on the Grid, you should use the DesiredCapabilities and the RemoteWebDriver objects.